EvanUtilService¶
Source | utils |
---|
Utility service for the whole angular core module.
devMode
-boolean
: isdevMode
enabled?isXXL
-boolean
: is browser width greater than 1500?isXL
-boolean
: is browser width greater than 1200?isLG
-boolean
: is browser width greater than 992?isMD
-boolean
: is browser width greater than 768?isSM
-boolean
: is browser width greater than 576?screenSize
-number
: current window sizelogger
-Logger
:BCC logger
instance
fillDevEnvVariables¶
fillDevEnvVariables(full);
Prefill localStorage dev environment variables, so they can easily accessed by developers. (‘angular-dev-logs’, ‘bc-dev-logs’,) Without passing the “full” parameter only this variables will be filled:
angular-dev-logs
: enable logs for frontend debug logsbc-dev-logs
: enable log for blockchain-core dev logs
By using the full parameter, all availabe configuration parameters will be empty applied to localStorage:
evan-account
: current account is saved here to access it globally without any serviceevan-bc-root
: blockchain-core configuration for default business centerevan-ens-address
: blockchain-core configuration for the ens addressevan-ens-events
: blockchain-core configuration for ens eventhub addressevan-ens-mailbox
: blockchain-core configuration for ens mailbox addressevan-ens-profiles
: blockchain-core configuration for ens profile addressevan-ens-resolver
: blockchain-core configuration for ens contract resolver addressevan-ens-root
: blockchain-core configuration for root ens (default = ‘evan’)evan-language
: overwrite the current used language (values: ‘en’, ‘de’, ‘fr’)evan-mail-read
: list of mail address that were readed by the userevan-mail-read-count
: amount of mails that the user readedevan-profile-creation
: check if the user is within the profile creation, used to navigate again to onboarding when the user reloads the page during profile creationevan-provider
: current used login provider (internal / external)evan-small-toolbar
: check if the evan-split-pane component is within the small viewevan-terms-of-use
: has the user accepted the terms of use? (only for local checking, is also saved within blockchain)evan-test-password
: password that is used for testing, user gets automatically logged with this password.- DANGER: should not be used in production, its a big security leak by passing clear text passwords to localStorage
evan-vault
: encrypted vault of the current logged in userevan-web3-provider
: overwrite web3 connection url (default is ‘wss://testcore.evan.network/ws’)
Parameters¶
full
-object
(optional): check if all internal variables should enrolled too
Example¶
utilService.fillDevEnvVariables(true);
addTemporaryStyle¶
utilService.addTemporaryStyle(name, css);
Add a temporary css style to the document head. E.g. it is used to style an image within an alert dialog.
Parameters¶
name
-string
: Name for the style. Style is available under class : evan-img-${name}css
-string
: css definition
Example¶
this.utils.addTemporaryStyle(trimmedName, `
.evan-temporary-${trimmedName} {
min-width: 257px;
}
.evan-temporary-${trimmedName} .alert-img-container {
background-color: ${primaryColor};
}
.evan-temporary-${trimmedName} .alert-img-container .alert-img {
background-color: ${secondaryColor};
background-image: url("${definition.imgSquare}");
}
`);
removeTemporaryImageStyle¶
utilService.removeTemporaryImageStyle(name);
Remove a temporary style sheet from the dom.
Parameters¶
name
-string
: Name for the style. Style is available under ID : evan-img-${name}
Example¶
this.utilService.removeTemporaryImageStyle(name);
isMobile¶
utilService.isMobile();
Check if we are on a mobile device (no matter if ionic app or browser).
Returns¶
boolean
: True if mobile, False otherwise.
isNativeMobile¶
utilService.isNativeMobile();
Check if we are on a mobile device (check if cordova is available).
Returns¶
boolean
: True if native mobile, False otherwise.
isMobileIOS¶
utilService.isMobileIOS();
check if we are on a ios mobile device (no matter if ionic app or browser).
Returns¶
boolean
: True if mobile ios, False otherwise.
isMobileAndroid¶
utilService.isMobileAndroid();
check if we are on a android mobile device (no matter if ionic app or browser).
Returns¶
boolean
: True if mobile android, False otherwise.
timeout¶
utilService.timeout(ms);
Promise wrapper for setTimeout.
Parameters¶
ms
-number
: Milliseconds to wait
Returns¶
Promise
returns void
: resolved when timeout is done
Example¶
await this.utilService.timeout(500);
immediate¶
utilService.timeout();
Promise wrapper for setimmediate.
Returns¶
Promise
returns void
: solved when setImmediate callback is called
Example¶
await this.utilService.immediate();
uniqueArray¶
utilService.uniqueArray(a);
Remove duplicate values from an array.
Parameters¶
a
-Array<any>
: Input Array
Returns¶
Array<any>
: the unique array
windowSize¶
await utilSerivce.windowSize(callback);
Registers and window resize watcher
Parameters¶
callback
-Function
: callback is called when size has changed and one time by calling the function directly
Returns¶
Promise
returns Function
: Function to unsubscribe after the callback function was called the first time
Example¶
const unsubscribe = await utilService.windowSize((width) => {
console.log(width);
})
setTimeout(() => unsubscribe());
sendEvent¶
utilsService.sendEvent(name, data);
emits a window.dispatchEvent
Parameters¶
name
-object
: even namedata
-Function
(optional): data to send
Example¶
// will open side panel on small devices
utilService.sendEvent('toggle-split-pane')
onEvent¶
utilService.onEvent(name, func);
runs window.addEventListener and func is called when event was triggered
Parameters¶
name
-object
: event namefunc
-Function
: function that is called on event occurence
Returns¶
Function
: Function to unsubscribe
Example¶
utilService.onEvent('toggle-split-pane', () => {
console.log('side panel was toggled');
});
deepCopy¶
utilService.deepCopy(arguments);
Runs JSON.parse(JSON.stringify(obj)) to make an maximum deep copy. Be
Beaware: dont apply recursive objects!
Parameters¶
obj
-object
: object that should be cloned
Returns¶
any
: cloned object
Example¶
x = { a: 1, b: 2, c: 3 }
y = utilService.deepCopy(x);
y.a = 4;
y.b = 5;
y.c = 6;
console.log(x) // => { a: 1, b: 2, c: 3 }
console.log(y) // => { a: 4, b: 5, c: 6 }
getParentByClassName¶
utilService.getParentByClassName(element, className);
Searches relative to an element an parent element with a specific element class
Parameters¶
element
-any
: reference elementclassName
-string
: class name that should be searched
Returns¶
Element
: parent element that should be searched
Example¶
<div class="im-a-parent">
<div>
<div id="mainElement"></div>
</div>
</div>
const mainElement = document.getElementById('mainElement');
const parent = utilService.getParentByClassName(mainElement, 'im-a-parent');
getOffsetTop¶
utilService.getOffsetTop($parent, $element, offsetTop);
Gets the full offset top of an element relative to an container
Parameters¶
$parent
-Element
: Parent container where the offset of the child should be get$element
-any
: Element to retrieve offset top fromoffsetTop
-number
(default = 0): last offset top for recursive function
Returns¶
number
: offset
Example¶
<div class="im-a-parent">
<div>
<div id="mainElement"></div>
</div>
</div>
const mainElement = document.getElementById('mainElement');
const parent = utilService.getParentByClassName(mainElement, 'im-a-parent');
const offset = utilService.getOffsetTop(parent, mainElement);
scrollTo¶
utilService.scrollTo($container, direction, scrollTo);
Scroll a container horizontal / vertical smooth to a specific position
Parameters¶
$container
-Element
: element that should be scrolleddirection
-string
: horizontal / verticalscrollTo
-number
: position to scroll to
Example¶
// scroll to most top position
utilService.scrollTo(container, 'horizontal', 0);
scrollUp¶
utilService.scrollUp($container, scrollTo, maxTurns);
Scrolls the suggestions container upwards
Parameters¶
$container
-Element
: $$container that should be scrolledscrollTo
-number
: where the container should be scrolled tomaxTurns
-number
(default): max turns to break animation (max. 200px)
Example¶
// scroll to most top position
utilService.scrollUp(container, 0);
scrollDown¶
utilService.scrollDown($container, scrollTo, maxTurns);
Scrolls the suggestions container downwards
Parameters¶
$container
-Element
: $$container that should be scrolledscrollTo
-number
: where the container should be scrolled tomaxTurns
-number
(default): max turns to break animation (max. 200px)
Example¶
// scroll to most top position
utilService.scrollDown(container, 500);
scrollLeft¶
utilService.scrollLeft($container, scrollTo, maxTurns);
Scrolls the suggestions container to the left.
Parameters¶
$container
-Element
: $$container that should be scrolledscrollTo
-number
: where the container should be scrolled tomaxTurns
-number
(default): max turns to break animation (max. 200px)
Example¶
// scroll to most top position
utilService.scrollLeft(container, 500);
scrollRight¶
utilService.scrollRight($container, scrollTo, maxTurns);
Scrolls the suggestions container to the right.
Parameters¶
$container
-Element
: $$container that should be scrolledscrollTo
-number
: where the container should be scrolled tomaxTurns
-number
(default): max turns to break animation (max. 200px)
log¶
utilService.log(message, level);
Using BCC log function to handle a generalized loggin mechanism.
Parameters¶
message
-object
: message to loglevel
-Function
: level to log the message with
isDeveloperMode¶
utilSerivce.isDeveloperMode();
Check if the user enabled developer mode within profile configuration. Can be enabled using the profile DApp under settings. It opens the following functionalities:
- enable logging & reporting DApp
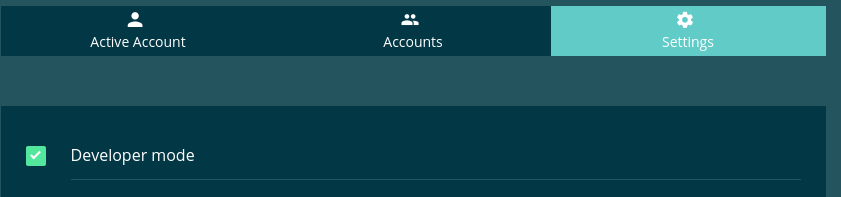
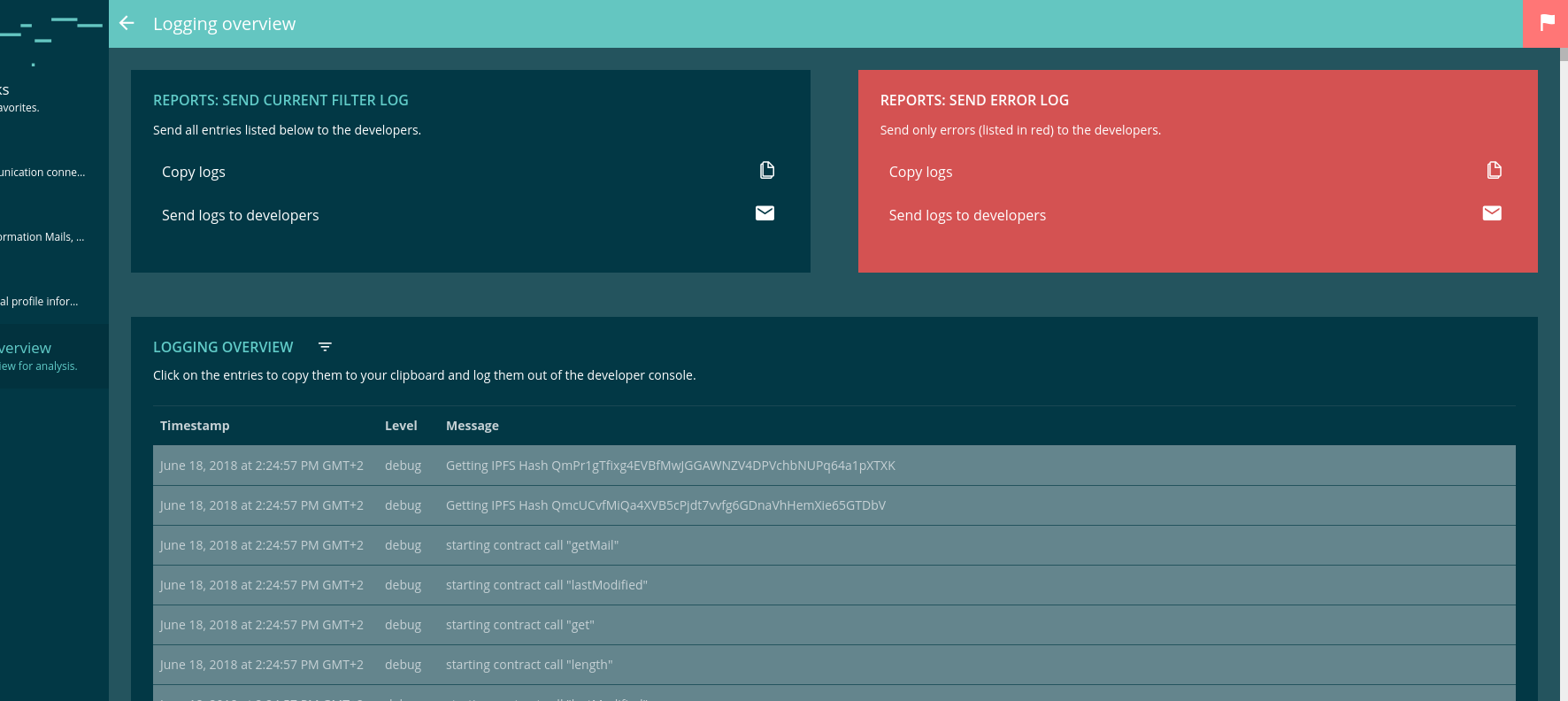
Returns¶
boolean
: True if developer mode, False otherwise.
notificationsEnabled¶
utilSerivce.notificationsEnabled();
Check if the user enabled notifications on the mobile devices.
Returns¶
boolean
: True if notifications are enabled, False otherwise.
getErrorLog¶
utilSerivce.getErrorLog(ex);
Transforms an Exception into an loggable string format. Returns the string if the exception is only a string.
Returns¶
string
: Transformed exception
Example¶
try {
throw new Error('Exception');
} catch (ex) {
this.core.utils.log(`Error : ${ this.utils.getErrorLog(ex) }`, 'error');
}
showLoading¶
utilSerivce.showLoading(context);
Shows the loading of a ref detached component
Parameters¶
context
-any
: this of the component